Commit 0fed7aa
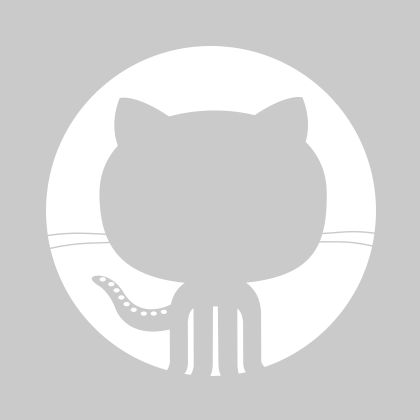
Hans-Kristian Arntzen
1 parent cce9bf5 commit 0fed7aa
File tree
5 files changed
+160
-7
lines changed5 files changed
+160
-7
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| 15 | + | |
15 | 16 |
| |
16 | 17 |
| |
17 | 18 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + |
+24-5
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
32 | 32 |
| |
33 | 33 |
| |
34 | 34 |
| |
35 |
| - | |
36 | 35 |
| |
37 | 36 |
| |
38 | 37 |
| |
39 | 38 |
| |
40 | 39 |
| |
41 | 40 |
| |
42 |
| - | |
43 |
| - | |
44 | 41 |
| |
45 | 42 |
| |
46 | 43 |
| |
| |||
426 | 423 |
| |
427 | 424 |
| |
428 | 425 |
| |
| 426 | + | |
| 427 | + | |
| 428 | + | |
| 429 | + | |
| 430 | + | |
| 431 | + | |
| 432 | + | |
| 433 | + | |
429 | 434 |
| |
430 | 435 |
| |
431 | 436 |
| |
| |||
434 | 439 |
| |
435 | 440 |
| |
436 | 441 |
| |
437 |
| - | |
| 442 | + | |
| 443 | + | |
| 444 | + | |
| 445 | + | |
438 | 446 |
| |
439 | 447 |
| |
440 | 448 |
| |
| |||
1882 | 1890 |
| |
1883 | 1891 |
| |
1884 | 1892 |
| |
| 1893 | + | |
| 1894 | + | |
| 1895 | + | |
| 1896 | + | |
| 1897 | + | |
| 1898 | + | |
| 1899 | + | |
| 1900 | + | |
1885 | 1901 |
| |
1886 | 1902 |
| |
1887 | 1903 |
| |
| |||
1891 | 1907 |
| |
1892 | 1908 |
| |
1893 | 1909 |
| |
1894 |
| - | |
| 1910 | + | |
| 1911 | + | |
| 1912 | + | |
| 1913 | + | |
1895 | 1914 |
| |
1896 | 1915 |
| |
1897 | 1916 |
| |
|
+2-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
10 | 10 |
| |
11 | 11 |
| |
12 | 12 |
| |
| 13 | + | |
13 | 14 |
| |
14 | 15 |
| |
15 | 16 |
| |
| |||
135 | 136 |
| |
136 | 137 |
| |
137 | 138 |
| |
138 |
| - | |
139 |
| - | |
140 | 139 |
| |
141 | 140 |
| |
142 | 141 |
| |
| |||
198 | 197 |
| |
199 | 198 |
| |
200 | 199 |
| |
| 200 | + | |
201 | 201 |
| |
202 | 202 |
| |
203 | 203 |
| |
|
0 commit comments