diff --git a/README.md b/README.md
index 40c4a6f5..ceca7afd 100644
--- a/README.md
+++ b/README.md
@@ -116,6 +116,7 @@ You can also override some of the default locale by `lang`.
| type | select the type of picker | date \|datetime\|year\|month\|time\|week | 'date' |
| range | if true, pick the range date | `boolean` | false |
| format | to set the date format. similar to moment.js | [token](#token) | 'YYYY-MM-DD' |
+| formatter | use your own formatter, such as moment.js | [object](#formatter) | - |
| value-type | data type of the binding value | [value-type](#value-type) | 'date' |
| default-value | default date of the calendar | `Date` | new Date() |
| lang | override the default locale | `object` | |
@@ -194,12 +195,12 @@ You can also override some of the default locale by `lang`.
| Unix Timestamp | X | 1360013296 |
| Unix Millisecond Timestamp | x | 1360013296123 |
-#### custom format
+#### formatter
-The `format` accepts an object to customize formatting.
+The `formatter` accepts an object to customize formatting.
```html
-
+
```
```js
@@ -207,13 +208,17 @@ data() {
return {
// Use moment.js instead of the default
momentFormat: {
- // Date to String
+ //[optional] Date to String
stringify: (date) => {
return date ? moment(date).format('LL') : ''
},
- // String to Date
+ //[optional] String to Date
parse: (value) => {
return value ? moment(value, 'LL').toDate() : null
+ },
+ //[optional] getWeekNumber
+ getWeek: (date) => {
+ return // a number
}
}
}
@@ -309,7 +314,6 @@ If you find this project useful, you can buy me a coffee
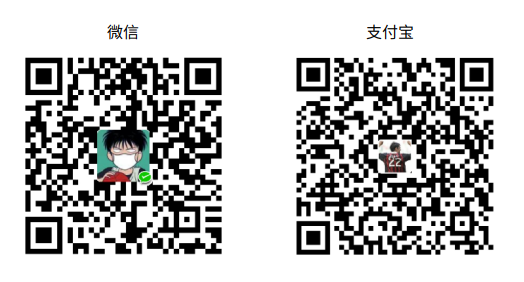
-
## License
[MIT](https://github.com/mengxiong10/vue2-datepicker/blob/master/LICENSE)
diff --git a/README.zh-CN.md b/README.zh-CN.md
index a0e9f503..30ab095b 100644
--- a/README.zh-CN.md
+++ b/README.zh-CN.md
@@ -114,6 +114,7 @@ import 'vue2-datepicker/locale/zh-cn';
| type | 日期选择的类型 | date \|datetime\|year\|month\|time\|week | 'date' |
| range | 如果是 true, 变成日期范围选择 | `boolean` | false |
| format | 设置格式化的 token, 类似 moment.js | [token](#token) | 'YYYY-MM-DD' |
+| formatter | 使用自己的格式化程序, 比如 moment.js | [object](#formatter) | - |
| value-type | 设置绑定值的类型 | [value-type](#value-type) | 'date' |
| default-value | 设置日历默认的时间 | `Date` | new Date() |
| lang | 覆盖默认的语音设置 | `object` | |
@@ -192,12 +193,12 @@ import 'vue2-datepicker/locale/zh-cn';
| Unix Timestamp | X | 1360013296 |
| Unix Millisecond Timestamp | x | 1360013296123 |
-#### custom format
+#### formatter
-`format` 接受一个对象去自定义格式化
+`formatter` 接受一个对象去自定义格式化
```html
-
+
```
```js
@@ -205,13 +206,17 @@ data() {
return {
// 使用moment.js 替换默认
momentFormat: {
- // Date to String
+ //[可选] Date to String
stringify: (date) => {
return date ? moment(date).format('LL') : ''
},
- // String to Date
+ //[可选] String to Date
parse: (value) => {
return value ? moment(value, 'LL').toDate() : null
+ },
+ //[可选] getWeekNumber
+ getWeek: (date) => {
+ return // a number
}
}
}
diff --git a/__test__/__snapshots__/date-picker.test.js.snap b/__test__/__snapshots__/date-picker.test.js.snap
index c235a459..55eb7379 100644
--- a/__test__/__snapshots__/date-picker.test.js.snap
+++ b/__test__/__snapshots__/date-picker.test.js.snap
@@ -92,7 +92,58 @@ exports[`DatePicker prop: clearable 1`] = `
`;
-exports[`DatePicker prop: custom format 1`] = `
+exports[`DatePicker prop: editable 1`] = `
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+`;
+
+exports[`DatePicker prop: formatter 1`] = `
@@ -580,54 +631,3 @@ exports[`DatePicker prop: custom format 1`] = `
`;
-
-exports[`DatePicker prop: editable 1`] = `
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-`;
diff --git a/__test__/date-picker.test.js b/__test__/date-picker.test.js
index ed5edfdc..30cf6e00 100644
--- a/__test__/date-picker.test.js
+++ b/__test__/date-picker.test.js
@@ -135,14 +135,14 @@ describe('DatePicker', () => {
expect(input.value).toBe('2019/10/10');
});
- it('prop: custom format', () => {
+ it('prop: formatter', () => {
wrapper = mount(DatePicker, {
propsData: {
valueType: 'format',
value: '13/10/2019',
open: true,
type: 'week',
- format: {
+ formatter: {
stringify(date) {
return format(date, 'dd/MM/yyyy');
},
diff --git a/src/date-picker.vue b/src/date-picker.vue
index 09493f63..a378e810 100644
--- a/src/date-picker.vue
+++ b/src/date-picker.vue
@@ -168,7 +168,7 @@ export default {
default: 'date',
},
format: {
- type: [String, Object],
+ type: String,
default() {
const map = {
date: 'YYYY-MM-DD',
@@ -181,6 +181,9 @@ export default {
return map[this.type] || map.date;
},
},
+ formatter: {
+ type: Object,
+ },
range: {
type: Boolean,
default: false,
@@ -347,6 +350,13 @@ export default {
},
},
},
+ created() {
+ if (typeof this.format === 'object') {
+ console.warn(
+ "[vue2-datepicker]: The prop `format` don't support Object any more. You can use the new prop `formatter` to replace it"
+ );
+ }
+ },
methods: {
handleClickOutSide(evt) {
const { target } = evt;
@@ -354,22 +364,28 @@ export default {
this.closePopup();
}
},
+ getFormatter(key) {
+ return (
+ (isObject(this.formatter) && this.formatter[key]) ||
+ (isObject(this.format) && this.format[key])
+ );
+ },
getWeek(date, options) {
- if (isObject(this.format) && typeof this.format.getWeek === 'function') {
- return this.format.getWeek(date, options);
+ if (typeof this.getFormatter('getWeek') === 'function') {
+ return this.getFormatter('getWeek')(date, options);
}
return getWeek(date, options);
},
parseDate(value, fmt) {
- if (isObject(this.format) && typeof this.format.parse === 'function') {
- return this.format.parse(value, fmt);
+ if (typeof this.getFormatter('parse') === 'function') {
+ return this.getFormatter('parse')(value, fmt);
}
const backupDate = new Date();
return parse(value, fmt, { locale: this.locale.formatLocale, backupDate });
},
formatDate(date, fmt) {
- if (isObject(this.format) && typeof this.format.stringify === 'function') {
- return this.format.stringify(date, fmt);
+ if (typeof this.getFormatter('stringify') === 'function') {
+ return this.getFormatter('stringify')(date, fmt);
}
return format(date, fmt, { locale: this.locale.formatLocale });
},